So when a new version of any OS comes out, I like to do a clean install. On Macs, this has usually been fairly easy, since HFS+ partitions are pretty flexible — they’ll let you add/remove/resize without a lot of hassle.
Until Yosemite.
In the past, I’ve made a new partition on my drive, installed the new OS X (like Mavericks), copied all my files from the old partition to the new one. Once I’m sure that all is fine and dandy, I will go back and delete the older partition and give the space to the new one.
But when I tried this with 10.10 Yosemite, I ran into a new problem. Partitions were no longer flexible. I couldn’t delete or resize any of them. I found this out after spending a full day getting the new Yosemite partition just right.
Some research found that Yosemite will change its partition from HFS+ to “Core Storage”. (According to Ars Technica, there’s not a clear reason why, either.) I’m not sure of all the technical details, but this apparently killed flexibility. So my new Yosemite install was stuck on a partition with only 10GB of free space, when it could have had 400 from the old Mavericks partition. It wouldn’t even let me delete the Mavericks partition. Everything was locked.
Fortunately, I found a solution. You can convert Yosemite’s Core Storage partition back to HFS+. Once I did that, Disk Utility worked fine again. Some notes:
- This is not destructive. You won’t lose anything.
- This only works if you have not encrypted the partition with FileVault.
Here’s how:
First, you have to get something called a lvUUID for the partition. (Don’t worry about the annoying acronym. This is all scarier than it looks.)
Open Terminal, and run this (you can copy/paste):
diskutil cs list
This will give you a list of partitions similar to this:
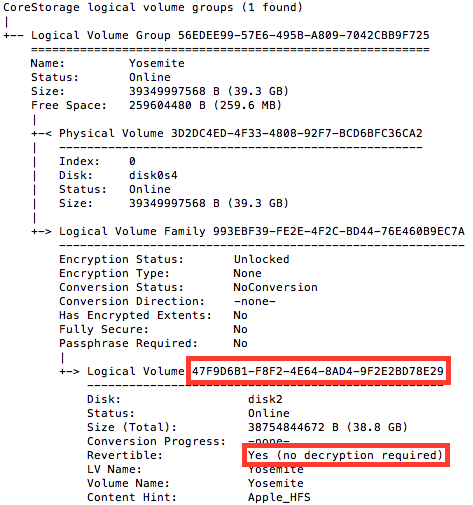
Okay. See how it says “Revertible: Yes”? That means we can convert it back. You’ll need that super-long string of letters/numbers. In the same Terminal window, type:
diskutil coreStorage revert [THAT LONG STRING OF STUFF]
So in this particular case, we would run:
diskutil coreStorage revert 47F9D6B1-F8F2-4E64-8AD4-9F2E2BD78E29
The results should be quick. Only seconds. Once that was finished, I was able to delete the Mavericks partition and resize my new Yosemite partition to fill all available space.
Some final notes:
- For more details, check out this forum thread on MacRumors. In particular, post #38.
- The first terminal command, diskutil cs list will only work if you actually have a CoreStorage partition.
- You can run this while booted into the Yosemite partition in question. No need to boot the recovery partition or anything.
- As I understand it, Apple’s Fusion Drives require Core Storage so this won’t work on those.