So you have a form in your app, and you want to tab from an NSTextField to an NSPopUpButton. You know, like any normal HTML form would using the tabindex attribute.
Well, you could use the dandy nextKeyView property, which lets you define which input should get focus next after tabbing. You could do it like this:
class RightViewController: NSViewController {
@IBOutlet var rageTextField: NSTextField!
@IBOutlet var furyPopup: MyNSPopUpButton!
override func viewDidLoad() {
super.viewDidLoad()
self.rageTextField.nextKeyView = furyPopup
}
}
It won’t work. It’ll work fine between NSTextFields and other stuff, but not your NSPopUpButton. Why? Well, [INSERT INCOHERENT SEETHE-RANTING HERE]. That’s why.
Look, the short explanation is that with NSPopUpButtons, canBecomeKeyView is set to false. Fix it by overriding using a subclass.
class MyNSPopUpButton: NSPopUpButton {
override var canBecomeKeyView: Bool {return true} // @&$('#$')!!
}
class ViewController: NSViewController {
@IBOutlet var rageTextField: NSTextField!
@IBOutlet var furyPopup: MyNSPopUpButton! // <-- LOOK AT THE "MY"
override func viewDidLoad() {
super.viewDidLoad()
print("(furyPopup.canBecomeKeyView)") // should be true
self.rageTextField.nextKeyView = furyPopup // should work now
}
}
Wait, one more thing. Don’t forget to change the popup’s class in IB, too:
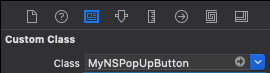